Adding related data with no-code backend REST API
In this section, we will update the Tasks page.
Go to TODOLISTS service, open the Tasks model, switch to the Integration tab.
Copy JavaScript code from the Create (POST) tab.
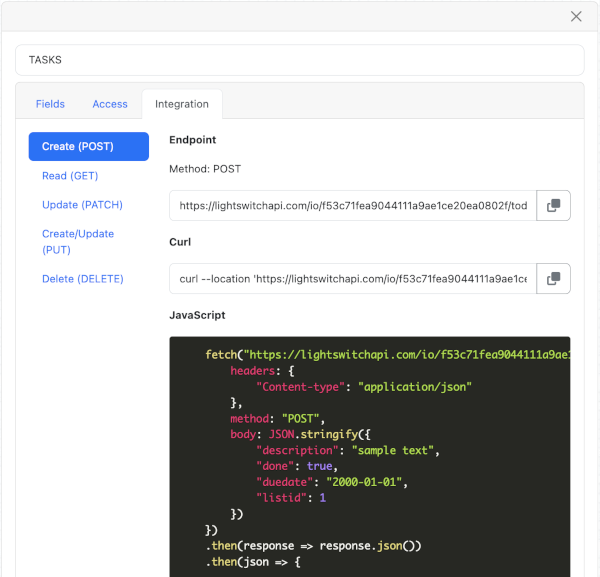
Go back to sample code and open the file src/components/Tasks/Tasks.js. Locate the function addTask().
// Add Task
const addTask = (newTask) => {
setLoading(true)
// ** INSERT CREATE CODE HERE **
// ** INSERT CREATE CODE ENDS **
}
Paste the JavaScript code from earlier inside the marked area.
// Add Task
const addTask = (description) => {
setLoading(true)
// ** INSERT CREATE CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]", {
headers: {
"Authorization": "Bearer " + "/**** INSERT token.value HERE ****/",
"Content-type": "application/json"
},
method: "POST",
body: JSON.stringify({
/********** REPLACE ***********
"description": "sample string",
"done": true,
"listid": [record id]
*******************************/
})
})
.then(response => response.json())
.then(json => {
if (!json.success)
throw new Error(json.message)
/****** HANDLE RESPONSE ********
console.log(json.data.id)
*******************************/
})
.catch((error) => {
// handle error
alert(error.message)
})
// ** INSERT CREATE CODE ENDS **
}
Update the Authorization header to include the authorization token. If you don't see the authorization header in sample code, make sure you've configured permissions as described in configuring permissions.
"Authorization": "Bearer " + auth.token?.value,
Next, we will update the body of the request.
body: JSON.stringify({
description: description,
done: false,
listId: listId
})
We'll also add some code to handle the response.
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// update state to display the new record
setTasks([...tasks, {id: json.data.id, description: description, done: false, listId: listId}])
// ***** UPDATED ***** //
})
The final output should look something like this.
// Add Task
const addTask = (description) => {
setLoading(true)
// ** INSERT CREATE CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]", {
headers: {
// ***** UPDATED ***** //
"Authorization": "Bearer " + auth.token?.value,
"Content-type": "application/json"
},
method: "POST",
body: JSON.stringify({
// ***** UPDATED ***** //
description: description,
done: false,
listId: listId
})
})
.then(response => response.json())
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// ***** UPDATED ***** //
// update state to display the new record
setTasks([...tasks, {id: json.data.id, description: description, done: false, listId: listId}])
})
.catch((error) => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
// handle error
alert(error.message)
})
// ** INSERT CREATE CODE ENDS **
}
Save your code. You should now be able to open any list in the app, and start adding tasks.
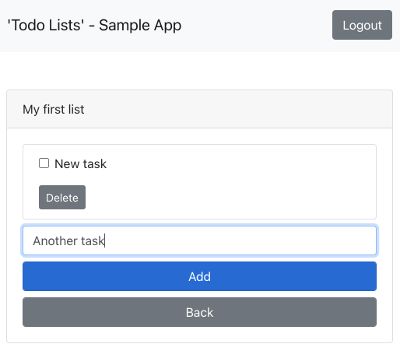