Filtering data with no-code backend REST API
Now we will retrieve saved tasks. When you open a list, you only want to see tasks that belong to it.
Go to TODOLISTS service, open the Tasks model, switch to the Integration tab.
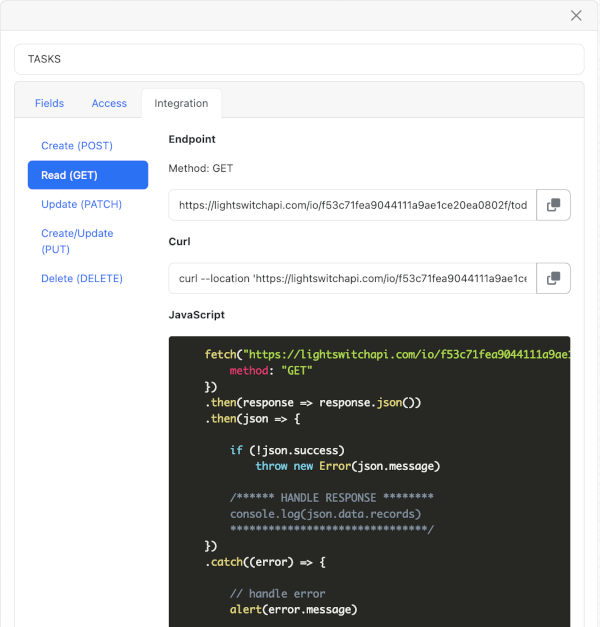
Copy JavaScript code from the Read (GET) tab.
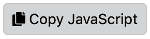
Go back to sample code and open the file src/components/Tasks/Tasks.js. Locate the function fetchTasks().
// Fetch Task
const fetchTasks = (listId) => {
setLoading(true)
// ** INSERT READ CODE HERE **
// ** INSERT READ CODE ENDS **
}
Paste the JavaScript code from earlier inside the marked area.
fetch("[YOUR LIGHTSWITCH API ENDPOINT]", {
headers: {
"Authorization": "Bearer " + "/**** INSERT token.value HERE ****/"
},
method: "GET"
})
.then(response => response.json())
.then(json => {
if (!json.success)
throw new Error(json.message)
/****** HANDLE RESPONSE ********
console.log(json.data.records)
*******************************/
})
.catch((error) => {
// handle error
alert(error.message)
})
Update the Authorization header to include the authorization token. If you don't see the authorization header in sample code, make sure you've configured permissions as described in configuring permissions.
"Authorization": "Bearer " + auth.token?.value
Next, we'll modify the URL of the API slightly to filter tasks by list ID.
Append a filter expression to the URL, as shown below.
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + "?$filter=LISTID eq " + listId, {
Add code to handle the response.
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// update state with data retrieved from server
setTasks(json.data.records);
// ***** UPDATED ***** //
})
The final output should look something like this.
// Fetch tasks
const fetchTasks = (listId) => {
// ** INSERT READ CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + "?$filter=LISTID eq " + listId, {
headers: {
"Authorization": "Bearer " + auth.token?.value,
},
method: "GET"
})
.then(response => response.json())
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// update state with data retrieved from server
setTasks(json.data.records);
// ***** UPDATED ***** //
})
.catch((error) => {
// handle error
alert(error.message)
})
// ** INSERT READ CODE ENDS **
}
Save your code and reload the app. You should now be able to open a list and see tasks belonging to it.
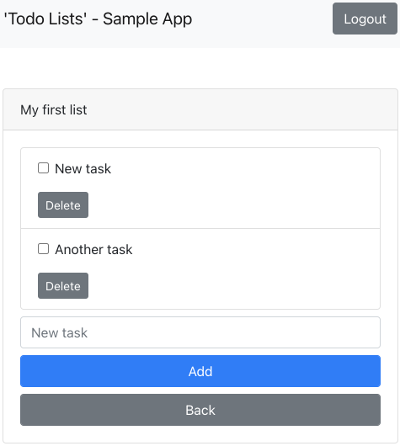