Reading data with no-code backend REST API
Go to TODOLISTS service, open the Lists model, switch to Integration.
Copy JavaScript code from the Read (GET) tab.
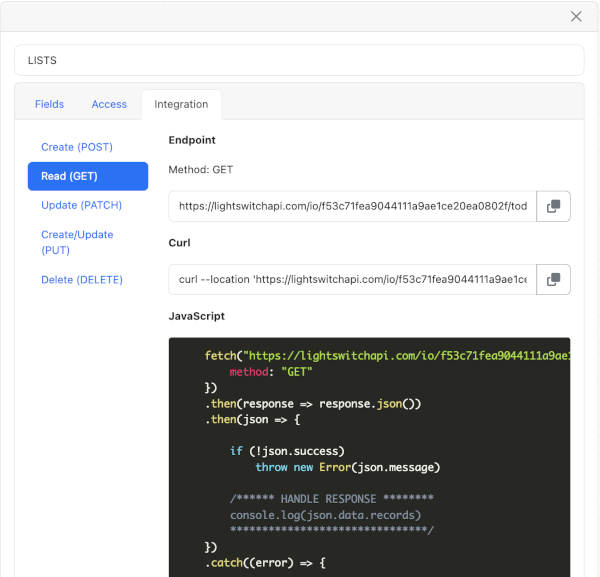
Go back to sample code and open the file components/Lists/Lists.js. Locate the function fetchLists().
// Fetch Lists
const fetchLists = () => {
setLoading(true)
setErrorMessage('')
// INSERT READ CODE HERE
// INSERT READ CODE ENDS
}
Paste the JavaScript code from earlier inside the marked area.
// Fetch Lists
const fetchLists = () => {
setLoading(true)
setErrorMessage('')
// INSERT READ CODE HERE
fetch("[YOUR LIGHTSWITCH API ENDPOINT]", {
method: "GET"
})
.then(response => response.json())
.then(json => {
if (!json.success)
throw new Error(json.message)
/****** HANDLE RESPONSE ********
console.log(json.data.records)
*******************************/
})
.catch((error) => {
// handle error
alert(error.message)
})
// INSERT READ CODE ENDS
}
Update the Authorization header to include the authorization token. If you don't see the authorization header in sample code, make sure you've configured permissions as described in configuring permissions.
"Authorization": "Bearer " + auth.token?.value
Unless an operation is given Public access, it's necessary to pass an Authorization header with any request.
Add code to handle the response.
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false);
// throw error if LightSwitch API returns an error response
if (!json.success)
throw new Error(json.message)
// update state with data retrieved from server
setLists(json.data.records);
// ***** UPDATED ***** //
})
The final output should look something like this.
// Fetch Lists
const fetchLists = () => {
setLoading(true)
setErrorMessage('')
// ** INSERT READ CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]", {
headers: {
"Authorization": "Bearer " + auth.token?.value
},
method: "GET"
})
.then(response => response.json())
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// ***** UPDATED ***** //
// update state with data retrieved from server
setLists(json.data.records);
})
.catch((error) => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
// handle error
alert(error.message)
})
// ** INSERT READ CODE ENDS **
}
Save your code and reload the app. You should see all the lists that you created previously.
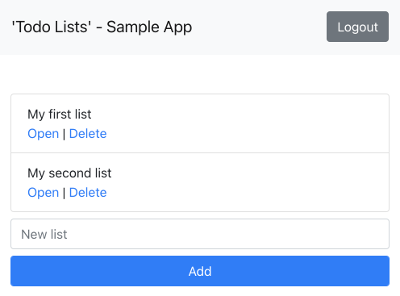