Deleting data with no-code backend REST API
In this step, we are looking at deleting records via the no-code backend.
After this step, users will be able to delete tasks.
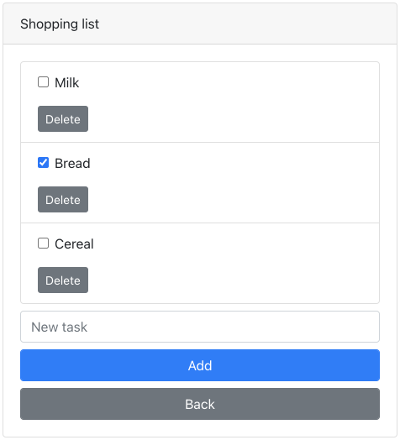
Go to TODOLISTS service, open the Tasks model, switch to the Integration tab.
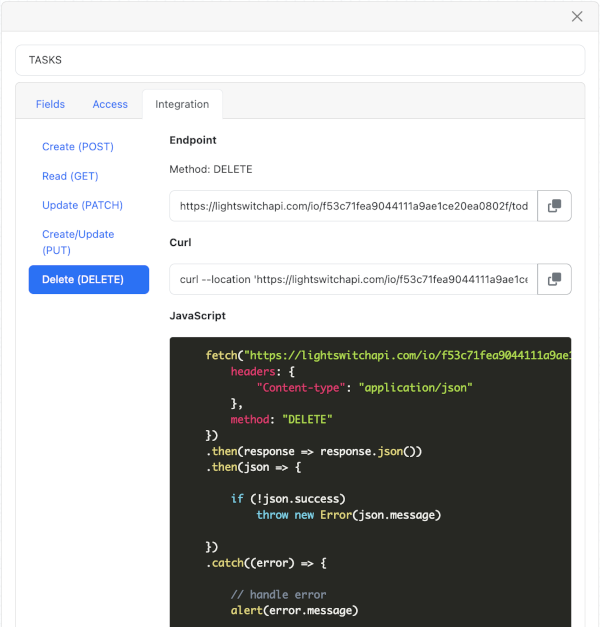
Copy JavaScript code from the Delete (DELETE) tab.
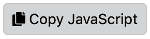
Go back to sample code and open the file src/components/Tasks/Tasks.js. Locate the function deleteTask().
// Delete Task
const deleteTask = (id) => {
setLoading(true)
// ** INSERT DELETE CODE HERE **
// ** INSERT DELETE CODE ENDS **
}
Paste the JavaScript code from earlier inside the marked area.
// Delete Task
const deleteTask = (taskId) => {
setLoading(true)
// ** INSERT DELETE CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + "/**** INSERT id HERE ****/", {
headers: {
"Authorization": "Bearer " + "/**** INSERT token.value HERE ****/",
"Content-type": "application/json"
},
method: "DELETE"
})
.then(response => response.json())
.then(json => {
if (!json.success)
throw new Error(json.message)
})
.catch((error) => {
// handle error
alert(error.message)
})
// ** INSERT DELETE CODE ENDS **
}
Append taskId to the URL.
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + taskId, {
Update the Authorization header to include the authorization token. If you don't see the authorization header in sample code, make sure you've configured permissions as described in configuring permissions.
"Authorization": "Bearer " + auth.token?.value,
Next, we'll handle the response.
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// remove the task from the user interace
setTasks(tasks.filter((task) => task.id !== taskId))
// ***** UPDATED ***** //
})
The final output should look something like this.
// Delete Task
const deleteTask = (taskId) => {
setLoading(true)
// ** INSERT DELETE CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + taskId, {
headers: {
"Authorization": "Bearer " + auth.token?.value,
"Content-type": "application/json"
},
method: "DELETE"
})
.then(response => response.json())
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// ***** UPDATED ***** //
// remove the task from the user interace
setTasks(tasks.filter((task) => task.id !== taskId))
})
.catch((error) => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
// handle error
alert(error.message)
})
// ** INSERT DELETE CODE ENDS **
}
Save your code. You should now be able to delete tasks in the app.
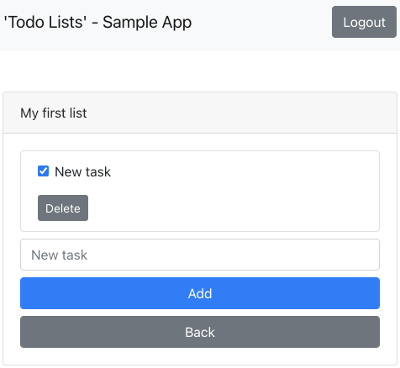