Updating data with no-code backend REST API
In this step, we will update existing data using the no-code backend REST API.
This would allow users of the app to mark an individual task as 'done'.
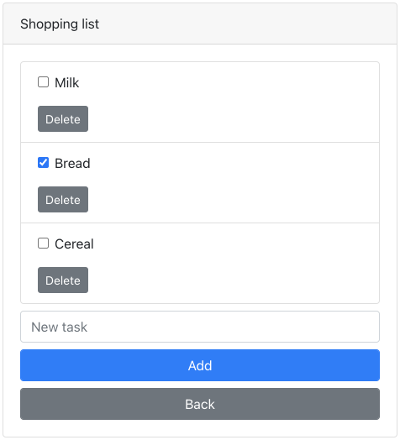
Go to TODOLISTS service, open the Tasks model, switch to the Integration tab.
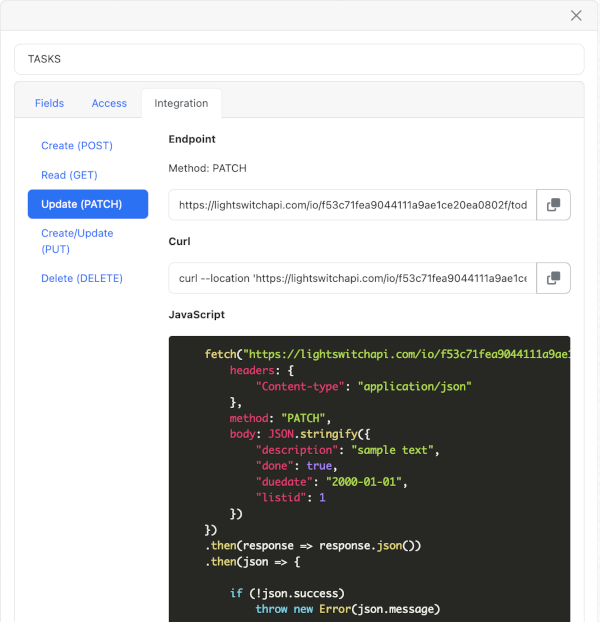
Copy JavaScript code from the Update (PATCH) tab.
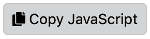
Go back to sample code and open the file src/components/Tasks/Tasks.js. Locate the function updateTask().
// Update Task
const updateTask = (taskId, taskDone) => {
// ** INSERT UPDATE CODE HERE **
// ** INSERT UPDATE CODE ENDS **
}
Paste the JavaScript code from earlier inside the marked area.
// Update Task
const updateTask = (taskId, taskDone) => {
// ** INSERT UPDATE CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + "/**** INSERT id HERE ****/", {
headers: {
"Authorization": "Bearer " + "/**** INSERT token.value HERE ****/",
"Content-type": "application/json"
},
method: "PATCH",
body: JSON.stringify({
/********** REPLACE ***********
description: "sample string",
done: true,
listid: id
*******************************/
})
})
.then(response => response.json())
.then(json => {
if (!json.success)
throw new Error(json.message)
})
.catch((error) => {
// handle error
alert(error.message)
})
// ** INSERT UPDATE CODE ENDS **
}
Append taskId variable to the URL.
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + taskId, {
Update the Authorization header to include the authorization token. If you don't see the authorization header in sample code, make sure you've configured permissions as described in configuring permissions.
"Authorization": "Bearer " + auth.token?.value,
Next, we will update the body of the request, passing in user input for the Done field.
body: JSON.stringify({
done: taskDone
})
Next, we will handle the response.
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// update state to reflect the new change
setTasks(tasks.map(function(task) { return task.id === taskId ? {...task, done: taskDone} : task; }))
// ***** UPDATED ***** //
})
The final output should look something like this.
// Update Task
const updateTask = (taskId, taskDone) => {
setLoading(true)
// ** INSERT UPDATE CODE HERE **
// ***** UPDATED ***** //
// added url param + taskId
fetch("[YOUR LIGHTSWITCH API ENDPOINT]" + taskId, {
headers: {
"Authorization": "Bearer " + auth.token?.value,
"Content-type": "application/json"
},
method: "PATCH",
body: JSON.stringify({
done: taskDone
})
})
.then(response => response.json())
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success)
throw new Error(json.message)
// ***** UPDATED ***** //
// update state to reflect the new change
setTasks(tasks.map(function(task) { return task.id === taskId ? {...task, done: taskDone} : task; }))
})
.catch((error) => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
// handle error
alert(error.message)
})
// ** INSERT UPDATE CODE ENDS **
}
Save your code and go back to the app. You should now be able to flag a task as done.
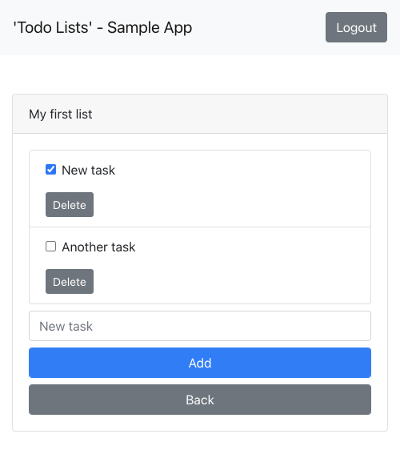