Integrating with no-code backend user authentication API
Open the downloaded source code in your favorite code editor. If you don't have one, we recommend Visual Studio Code.
Navigate to src/components/Account/Login.js.
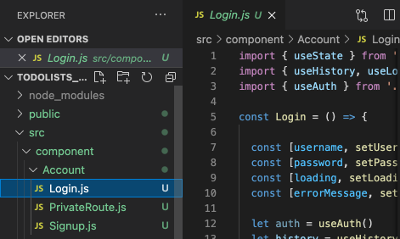
Scroll down to the onSubmit function, where we will make a change.
const onSubmit = (e) => {
e.preventDefault()
if (!validate())
return
setLoading(true)
// ** INSERT AUTHENTICATION CODE HERE **
// *************************************
}
If you already don't have it open, go to Services, and open the 'TODOLISTS' service that we created earlier.
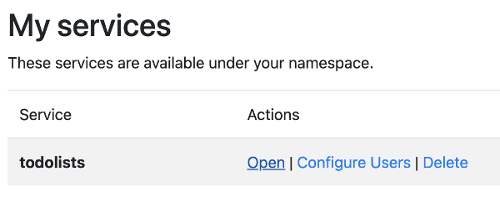
Open the Users model, and switch to the Integration tab.

Click on Authenticate.
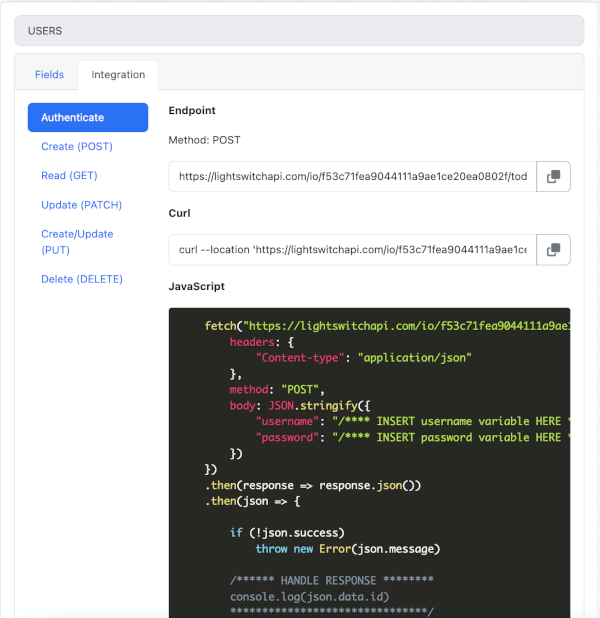
Below the JavaScript code, click Copy JavaScript
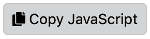
Go back to the file login.js, and paste the code where marked inside the onSubmit function.
const onSubmit = (e) => {
e.preventDefault()
if (!validate())
return
setLoading(true)
// ** INSERT AUTHENTICATION CODE HERE **
// ** INSERT AUTHENTICATION CODE ENDS **
}
const onSubmit = (e) => {
e.preventDefault()
if (!validate())
return
setLoading(true)
// ** INSERT AUTHENTICATION CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]", {
headers: {
"Content-type": "application/json"
},
method: "POST",
body: JSON.stringify({
username: username,
password: password
})
})
.then(response => response.json())
.then(json => {
if (!json.success)
throw new Error(json.message)
/****** HANDLE RESPONSE ********
console.log(json.data.id)
*******************************/
})
.catch((error) => {
// handle error
alert(error.message)
})
// *************************************
}
Update the JSON body of the request to pass username and password input.
body: JSON.stringify({
"username": username,
"password": password
})
Update the code that handles the response back from the API to save the authentication token locally.
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success){
throw new Error(json.message)
}
// ***** UPDATED ***** //
// save token and move to the requested location or main page
auth.setToken(json.data.token)
navigate(from)
})
The final output should look something like this.
const onSubmit = (e) => {
e.preventDefault()
if (!validate())
return
setLoading(true)
// ** INSERT AUTHENTICATION CODE HERE **
fetch("[YOUR LIGHTSWITCH API ENDPOINT]", {
headers: {
"Content-type": "application/json"
},
method: "POST",
body: JSON.stringify({
username: username,
password: password
})
})
.then(response => response.json())
.then(json => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
if (!json.success){
throw new Error(json.message)
}
// ***** UPDATED ***** //
// save token and move to the requested location or main page
auth.setToken(json.data.token)
navigate(from)
})
.catch((error) => {
// ***** UPDATED ***** //
// indicate that the action is complete
setLoading(false)
// handle error
alert(error.message)
});
}
Save your code. In the next step, we'll create an administrator account to test this code.